1. What is Python? What are the benefits of using Python language as a tool in the present scenario?
Python is a widely-used general-purpose, object-oriented, and high-level programming language. Python is commonly used for developing websites and software, task automation, data analysis, data visualization and machine learning.
The following are the benefits of using Python language:
- Object-Oriented Language
- High-Level Language
- Dynamically Typed language
- Extensive support Libraries like NumPy, Pandas, Matplotlib, TensorFlow etc.,
- Presence of third-party modules
- Free and Open source
- Versatile and Extensible
- Portable and Interactive
2. What are the different data types available in Python?
Python
supports various data types such as integers, floating-point numbers, strings,
booleans, lists, tuples, dictionaries, and sets. Each data type has its own
characteristics and use cases in programming.
1.
Numeric Types:
- int: Represents integer values without a fractional component, e.g., 10, -3, 42.
- float: Represents real numbers, i.e., numbers with a decimal point or in exponential form, e.g., 3.14, -0.001, 2e2.
- complex: Represents complex numbers, which include a real part and an imaginary part, e.g., 3+4j.
2.
Sequence Types:
- str (string): Represents a sequence of Unicode characters, e.g., “Hello, world!”.
- list: An ordered, mutable (changeable) collection of items that can be of mixed types, e.g., [1, “a”, 3.14].
- tuple: An ordered, immutable collection of items that can be of mixed types, e.g., (1, “a”, 3.14).
3.
Mapping Type:
- dict (dictionary): An unordered collection of items where each item is stored as a key-value pair. Keys must be unique and immutable, e.g., {“name”: “Alice”, “age”: 30}.
4.
Set Types:
- set: An unordered collection of unique elements, mutable, e.g., {1, 2, 3}.
- frozenset: An immutable version of a set, e.g., frozenset({1, 2, 3}).
5.
Boolean Type:
- bool: Represents two values: True or False, which are often the result of comparisons or conditions.
3. How do you declare and initialize variables in Python? Explain the rules and conventions for defining variables in Python.
In Python, variable names are identifiers that are used to store values in memory. In simple words, variables in Python are declared by assigning a value to them.
For example, x = 10 initializes a variable named x with a value of 10.
Rules for defining variable in Python:
- Variable names must start with a letter (a-z, A-Z) or an underscore (_).
- Variable names cannot start with number.
- After the first character, variable names can include letters, digits (0-9), or underscores.
- Variable names are case-sensitive. For example, myVariable, MyVariable, and myvariable are considered different variables.
- You cannot use Python keywords or reserved words as variable names. These include words like if, for, return, global, in, etc., as they hold special meaning in Python syntax.
- Variable names cannot contain special symbols like !, @, #, $, %, etc.
4. Explain the
difference between mutable and immutable data types in Python. Provide examples
of each.
Mutable
data types can be modified after creation, while immutable data types cannot.
For example, lists and dictionaries are mutable, meaning you can change their
elements or values. On the other hand, tuples and strings are immutable; once
they are created, their values cannot be altered.
For
instance:
Mutable
Example:
my_list = [1, 2, 3]
my_list.append(4)
# Modifying the list
print(my_list)
# Output: [1, 2, 3, 4]
Immutable
Example:
my_tuple = (1, 2, 3)
my_tuple[0] = 4
# Trying to modify the tuple
(Error: ‘tuple’ object does not support item
assignment)
5. What is
type casting in Python?
Type
casting, also known as type conversion, is the process of converting one data
type into another. In Python, you can use built-in functions to perform type
casting.
For
example,
Eg
1: int(5.6) #converts the float 5.6 into an integer, resulting in 5.
Eg
2:
num_int
= 7
num_float
= float(num_int)
print(num_float
/ 2) # Output: 3.5
6. What is the purpose of control flow in Python? Explain the different types of control flows used in python.
Control flow is used to dictate the order in which statements are executed in a program based on certain conditions or loops.
a) Conditional Statements (if, elif, else):
- Conditional statements are used to execute different blocks of code based on whether a certain condition is true or false.
- The if statement checks a condition and executes a block of code if the condition is true.
- The elif statement allows for additional conditions to be checked if the previous conditions were false.
- The else statement provides a block of code to execute if none of the previous conditions were true.
Example:
x = 10
if x > 5:
print(“x is greater than 5”)
elif x == 5:
print(“x is equal to 5”)
else:
print(“x is less than 5”)
b) Loops (for, while):
- Loops allow you to execute a block of code repeatedly.
- The for loop iterates over a sequence (such as a list, tuple, or string) or any iterable object.
- The while loop continues to execute a block of code as long as a specified condition is true.
# Example:
fruits = [“apple”, “banana”, “cherry”]
for fruit in fruits:
print(fruit)
In the for loop example, the loop iterates over each element in the fruits list and prints it.
# Example of while loop
x = 0
while x < 5:
print(x)
x += 1
In the while loop example, the loop prints the value of x as long as it is less than 5.
c) Break and Continue Statements:
- These statements can be used within loops to control the flow of execution.
- The break statement terminates the loop prematurely when a certain condition is met.
- The continue statement skips the rest of the code inside the loop for the current iteration and proceeds to the next iteration.
# Example:
for i in range(10):
if i == 5:
break # exits the loop when i equals 5
print(i)
for i in range(10):
if i % 2 == 0:
continue # skips even numbers
print(i)
- In the first for loop example, the loop exits prematurely when i equals 5 due to the break statement.
- In the second for loop example, the loop skips even numbers using the continue statement, printing only odd numbers.
d) Function Calls: Functions in Python allow for a modular approach to programming by enabling reusable pieces of code. A function is defined with the def keyword, and when called, the statements within it are executed.
8. How do you use the “break” and “continue” statements in loops?
The break and continue statements in Python are used to alter the flow of a loop (for or while). They both are used inside loops and have different purposes:
Break Statement:
- The break statement is used to prematurely exit a loop when a certain condition is met. It allows you to terminate the loop’s execution before it reaches its natural end.
- Typically used when a specific condition is met during the execution of the loop, and there’s no need to continue iterating. For example, searching for a particular item in a list and stopping the loop once the item is found.
- Break statement terminates the loop’s execution entirely and moves the program control to the line immediately following the loop
Example:
for num in range(1, 10):
if num == 5:
break # Exit the loop when num is 5
print(num)
# Output: 1 2 3 4
Continue Statement:
- When a specific condition is met, and you want to skip to the next iteration of the loop without executing the rest of the code block for the current iteration. It’s often used to avoid specific values or conditions within a loop.
- Skips the rest of the code inside the loop for the current iteration and continues with the next iteration of the loop.
Example:
for num in range(1, 10):
if num == 5:
continue # Skip the rest of the loop for num 5
print(num)
# Output: 1 2 3 4 6 7 8 9
Key Differences between Break and Continue Statement
Loop Exit vs. Iteration Skip: break exits the loop completely, while continue skips the current loop iteration and proceeds with the next one.
8. How can
you iterate over both the index and elements of a list using a “for”
loop? Provide an example.
To iterate
over both the index and elements of a list in Python, you can use the
enumerate() function.
enumerate()
adds a counter to an iterable and returns it in a form of enumerating object.
This enumerating object can then be used directly in for loops to get both the
index and the value of each item in the list.
Here’s an
example:
my_list =
[‘apple’, ‘banana’, ‘cherry’]
for index,
element in enumerate(my_list):
print(index, element)
In this example, my_list contains three strings. The enumerate() function is used in the for loop to get both the index (index) and the value (element) of each item in the list.
The output of this code would be:
0 apple
1 banana
2 cherry
9. What is a function in Python?
In Python, a function is a reusable block of code that performs a specific task or a set of tasks. Functions provide modularity and abstraction, allowing you to break down your code into smaller, manageable pieces. By defining functions, you can organize your code, improve readability, and promote code reuse.
Functions in Python can be categorized into two types:
- Built-in Functions: These are functions that are provided by Python itself, such as print(), len(), max(), min(), etc. They are available for use without needing to define them.
- User-defined Functions: These are functions that you define yourself to perform custom tasks. You can create user-defined functions to encapsulate specific functionalities and then call them whenever needed.
10. How do you define a function in Python?
In Python, you can define a function using the def keyword followed by the function name, parentheses (), and a colon :. Any parameters that the function takes are specified within the parentheses. The body of the function, containing the code to be executed, is indented.
Syntax of defining a function:
def greet():
print(“Hello, world!”)
In this example:
- def keyword indicates the start of a function definition.
- greet is the name of the function.
- () specifies that the function doesn’t take any parameters.
- print(“Hello, world!”) is the code inside the function body.
Once the function is defined, you can call it by using its name followed by parentheses. For example:
greet() # Output: Hello, world!
When this line is executed, the code inside the greet() function is executed, and “Hello, world!” is printed to the console. Defining and using functions is a fundamental aspect of Python programming, enabling you to create modular and reusable code for various tasks. Functions help make your code more organized, efficient, and easier to maintain.
11. What is the difference between arguments and parameters in Python?
In Python, “parameters” and “arguments” are related terms used in the context of functions, but they have different meanings:
Parameters:
- Parameters are placeholders defined in the function signature.
- They are used to specify what kind of information a function needs to perform its task.
- Parameters are essentially variables that represent the data that a function expects to receive.
- Parameters are defined when the function is declared.
- Parameters are listed within the parentheses following the function name.
Example:
def add (a, b): # ‘a’ and ‘b’ are parameters
return a + b
Arguments:
- Arguments are the actual values passed to a function when it is called.
- They are the concrete values that are supplied to the function to satisfy the parameters.
- Arguments are provided when the function is invoked, and they are passed inside the parentheses.
- The number of arguments passed must match the number of parameters declared in the function signature.
Example:
Result = add (3,5) #3 and 5 are arguments
12. What is a decorator in Python?
A decorator in Python is a higher-order function that allows you to extend or modify the behavior of another function or method without modifying its source code directly. Decorators provide a clean and concise way to add functionality to existing functions or methods by wrapping them with additional code. They are particularly useful for implementing cross-cutting concerns such as logging, authentication, caching, or performance monitoring.
Purpose of Decorators:
- Code Reusability: Decorators promote code reusability by allowing you to define reusable functionality that can be applied to multiple functions or methods.
- Separation of Concerns: Decorators help separate the core logic of a function from auxiliary concerns such as logging, caching, or error handling, leading to cleaner and more maintainable code.
- Promoting Single Responsibility Principle (SRP): By delegating additional responsibilities to decorators, you can adhere to the SRP, ensuring that each function or method focuses on a single task or responsibility.
- Flexibility and Extensibility: Decorators enable you to easily add or remove functionality from functions or methods without modifying their source code directly, providing flexibility and extensibility to your codebase.
13. Provide an example of decorator and explain its purpose.
Let’s create a decorator my_decorator that simply prints a message before and after the execution of a function it decorates.
def
my_decorator(func):
def wrapper():
print(“Something is happening
before the function is called.”)
func()
print(“Something is happening
after the function is called.”)
return wrapper
#
Use the decorator
@my_decorator
def
say_hello():
print(“Hello!”)
#Calling the function
say_hello()
Output
Something
is happening before the function is called.
Hello!
Something
is happening after the function is called.
How
It Works
- my_decorator is a function that takes another function func as an argument.
- Inside my_decorator, the wrapper function is defined. It wraps the behavior of the func function: it prints a message, then calls func itself, and then prints another message.
- my_decorator returns the wrapper function as its result.
- To apply the decorator, you use the @ syntax before the definition of the function to be decorated (say_hello). This is syntactic sugar for say_hello = my_decorator(say_hello).
14. What is a
list in Python? How do you create and manipulate lists?
In Python, a list is a versatile and commonly used data structure that represents an ordered collection of elements. Lists are mutable, meaning they can be modified after creation. Lists can contain elements of different data types, including integers, floats, strings, and even other lists.
Creating Lists: Lists are created using square brackets [], with elements separated by commas.
Here’s how
you create a simple list:
my_list = [1, 2, 3, ‘a’, ‘b’, ‘c’]
In this example, my_list contains integers (1, 2, 3) and strings (‘a’, ‘b’, ‘c’).
Lists can
also be empty.
Manipulating Lists:
Once a list
is created, you can manipulate it in various ways using built-in methods. Here
are some common methods for list manipulation:
· Appending Elements: The append() method adds an element to the end of the list.
· Extending Lists: The extend() method adds elements from another iterable (such as another list) to the end of the list.
· Inserting Elements: The insert() method inserts an element at a specified index in the list.
· Removing Elements: The remove() method removes the first occurrence of a specified element from the list.
· Popping Elements: The pop() method removes and returns the element at a specified index. If no index is provided, it removes and returns the last element.
·
Indexing
and Slicing:
You can access elements in a list using indexing and slicing.
15. What is a
tuple in Python?
In Python, a tuple is a data structure similar to a list but with one key difference: tuples are immutable, while lists are mutable.
Definition:
- A tuple is an ordered collection of elements, enclosed within parentheses ().
- Elements in a tuple can be of any data type, including integers, floats, strings, tuples, or even other data structures.
- Tuples maintain the order of elements, meaning the order in which elements are defined is preserved when accessing them.
Creating Tuples: Tuples are created using parentheses () with elements separated by commas.
Here’s an
example of creating a tuple:
my_tuple = (1, 2, 3,
‘a’, ‘b’, ‘c’)
16. Tell me
the difference between a Tuple and a List:
Mutability:
·
Tuple: Tuples are immutable,
meaning once a tuple is created, its elements cannot be changed, added, or
removed.
·
List: Lists are mutable,
allowing you to modify their elements after creation. Elements can be added,
removed, or modified in a list.
Syntax:
·
Tuple: Tuples are defined
using parentheses ().
·
List: Lists are defined using
square brackets [].
Performance:
·
Tuple: Tuples are generally
more memory-efficient and faster to access compared to lists because of their
immutability.
·
List: Lists may be less
efficient in terms of memory and performance, especially when dealing with
large datasets or frequent modifications.
Use
Cases:
·
Tuple: Tuples are often used
for representing fixed collections of items, such as coordinates, database
records, or function return values.
·
List: Lists are preferred
when you need a dynamic collection of items that may change over time, such as
a list of tasks, user inputs, or intermediate results in a computation.
17. What is a
dictionary in Python? How do you create and access elements in a dictionary?
In Python, a
dictionary is a versatile and powerful data structure that represents an ordered
collection of key-value pairs. Dictionaries are commonly used for mapping keys
to values and are useful for organizing and accessing data based on unique
identifiers (keys) rather than indices. Each key in a dictionary must be unique
and immutable (such as strings, numbers, or tuples), while values can be of any
data type and can be duplicated.
Creating
a Dictionary:
Dictionaries are created using curly braces {} and key-value pairs
separated by colons: Here’s how you create a dictionary:
my_dict =
{‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
In this
example, my_dict is a dictionary with three key-value pairs: ‘name’:
‘Alice’, ‘age’: 30, and ‘city’: ‘New York’.
Accessing
Elements in a Dictionary:
Elements in a dictionary are accessed using square brackets [] with the
key.
print(my_dict[‘name’])
# Output: Alice
Dictionary
are ordered collection of key-value pairs which means that items in a
dictionary are remembered in the order in which they were inserted.
Example:
my_dict =
{‘one’: 1, ‘two’: 2, ‘three’: 3}
# Adding an
item
my_dict[‘four’]
= 4
for key in
my_dict:
print(key, my_dict[key])
In this example, the
keys will be printed in the order they were added: ‘one’, ‘two’, ‘three’,
‘four’.
18. What are the characteristics of Dictionaries?
Key Characteristics of Dictionaries:
Unordered: Dictionaries are ordered collections which means that iteams in a dictionary are remembered in the order in which they were inserted.
Mutable: Dictionaries are mutable, so you can modify, add, or remove key-value pairs after creation.
Keys and Values: Keys must be unique and immutable, while values can be of any data type and can be duplicated.
Example of Modifying a Dictionary:
· my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
· my_dict[‘age’] = 35 # Modifying the value associated with the ‘age’ key
· my_dict[‘gender’] = ‘Female’ # Adding a new key-value pair
· del my_dict[‘city’] # Removing the ‘city’ key and its associated value
In this example, we modify the value associated with the key ‘age’, add a new key-value pair ‘gender’: ‘Female’, and remove the key ‘city’ and its associated value from the dictionary.
19. How do you access elements in a dictionary by key?
Elements in a dictionary are accessed using square brackets [] with the key.
Example:
my_dict =
{‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
print(my_dict[‘name’])
#Output: Alice
20. What is a set in Python?
In Python, a set is an unordered collection of unique elements. Sets are mutable, meaning you can modify them after creation, but they do not allow duplicate elements. Sets are useful for operations like membership testing, removing duplicates from a sequence, and performing mathematical set operations such as union, intersection, difference, and symmetric difference.
Creating a Set: Sets are created using curly braces {} or the set() constructor. Elements are separated by commas ,
my_set = {1, 2, 3, 4, 5}
A = {1,2, 2, 3,4, 4, 5}
set(A) #Output = 1,2,3,4,5
21. How do you iterate over elements in a list, tuple, dictionary, and set in Python?
Explanation: Elements in a list, tuple, dictionary, and set can be iterated using loops such as for loop or using methods like iter().
Example:
for item in
my_list:
print(item)
for item in
my_tuple:
print(item)
for key,
value in my_dict.items():
print(key, value)
for item in my_set:
print(item)
22. What is the difference between append() and extend() methods in Python lists?
Explanation: The append() method adds a single element to the end of a list, while the extend() method adds multiple elements (from another iterable) to the end of a list.
Example:
my_list =
[1, 2, 3]
my_list.append(4) # [1, 2, 3, 4]
my_list.extend([5,
6]) # [1, 2, 3, 4, 5, 6]
23. What is file handling in Python? How do you open and close files?
File
Handling in Python: File
handling in Python refers to the process of working with files on the disk.
This includes tasks such as reading data from files, writing data to files, and
performing various operations like appending, deleting, or modifying data
within files.
Opening and Closing Files: In Python, files are opened using the open() function and closed using the close() method or by using a context manager with the with statement. Here’s how you open and close files:
1. Opening Files:
To open a
file, you use the open() function, specifying the filename and the mode
in which you want to open the file. The mode can be ‘r’ for reading, ‘w’
for writing (truncating the file if it already exists), ‘a’ for
appending, or ‘r+’ for both reading and writing.
file = open(‘example.txt’, ‘r’) # Opens the file in read mode
If the file does not exist, Python will raise a FileNotFoundError unless you open the file in write or append mode.
2. Closing Files:
It’s
essential to close files after you finish working with them to release system
resources. You can close a file using the close() method.
However, forgetting to close files can lead to resource leaks or issues with data consistency. To ensure files are closed properly, it’s recommended to use a context manager with the with statement.
with open(‘example.txt’, ‘r’) as file:
# Read data from file
data = file.read()
print(data)
24. How do you read and write data from a file in Python?
Data can be read from a file using various methods like read(), readline(), or readlines().
Example:
with open(‘example.txt’, ‘r’) as file:
data = file.read()
Data can be written to a file using the write() method or using context managers with the with statement.
Example:
with
open(‘example.txt’, ‘w’) as file:
file.write(“Hello, world!”)
25. Explain the difference between reading modes ‘r’, ‘w’, and ‘a’ in Python file handling.
‘r’: Opens a file for reading (default mode). Raises an error if the file does not exist.
‘w’: Opens a file for writing. Creates a new file or truncates an existing file.
‘a’: Opens a file for appending. Cretae a new file if it does not exist or appends to an existing file.
26. How do you handle exceptions when working with files in Python?
File-related exceptions such as FileNotFoundError and PermissionError can be handled using try-except blocks.
Example:
try:
with open(‘example.txt’, ‘r’) as file:
data = file.read()
except
FileNotFoundError:
print(“File not found”)
27. What is exception handling in Python? Why is it important?
Exception handling in Python refers to the process of managing and responding to errors or exceptional conditions that occur during the execution of a program. When an error occurs in a Python program, it raises an exception, which can disrupt the normal flow of the program if not handled properly. Exception handling allows programmers to anticipate and gracefully handle these exceptional situations, preventing the program from crashing and providing a way to recover from errors.
Let us understand why Exception Handling is Important
Preventing Program Crashes: Exception handling prevents programs from crashing abruptly when errors occur, ensuring that the program can gracefully recover from unexpected conditions.
Improving Code Robustness: By handling exceptions, programmers can write more robust and reliable code that can withstand unexpected inputs or conditions.
Debugging and Error Reporting: Exception handling provides a mechanism for identifying and reporting errors, making it easier to debug and troubleshoot problems in the code.
Enhancing User Experience: Handling exceptions allows programs to provide meaningful error messages or take appropriate actions when errors occur, enhancing the overall user experience.
Maintaining Program Stability: Properly handling exceptions helps maintain the stability and integrity of the program, ensuring that it continues to function correctly under various conditions.
Exception in python are erros that occurs during program execution, disrupting the normal flow of the program. Example include ZeroDivisionError, TypeError, ValueError, FileNotFoundError etc.
28. How do you
raise custom exceptions in Python?
·
You
can raise custom exceptions using the raise keyword followed by the type
of exception and an optional error message.
x = 10
if x > 5:
raise ValueError(“x should not be greater than 5”)
· The assert statement is used for debugging purposes to check conditions that should always be true. If the condition is false, an AssertionError exception is raised.
Example:
x = 10
assert x > 5, “x should be greater than 5”
· File-related exceptions such as FileNotFoundError or PermissionError can be handled using try-except blocks when performing file operations.
Example:
try:
file = open(“example.txt”, “r”)
# File operations…
except FileNotFoundError:
print(“File Not Found”)
29. What is Object-Oriented Programming (OOP)?
Object-Oriented Programming (OOP) is a programming paradigm that organizes code into objects, which are instances of classes. It is based on the concept of “objects,” which can contain data in the form of fields (also known as attributes or properties) and code in the form of procedures (methods or functions). OOP focuses on modeling real-world entities as objects and their interactions.
In OOP, each object is an instance of a class, which serves as a blueprint or template for creating objects. Classes define the structure and behavior of objects by encapsulating data and methods that operate on the data. This encapsulation promotes modularity, reusability, and maintainability of code.
30. Name the characteristics of object-oriented programming?
Modularity: OOP allows code to be organized into modular units (classes and objects), making it easier to manage and maintain large codebases. Each class represents a distinct module with its own data and behavior.
Reusability: OOP promotes code reuse through inheritance and composition. Classes can inherit properties and methods from other classes, allowing developers to reuse existing code and extend functionality.
Abstraction: OOP allows developers to model real-world entities as objects with well-defined interfaces. This abstraction hides the implementation details of objects, allowing users to interact with objects at a higher level of abstraction.
Encapsulation: OOP encapsulates data and behavior within objects, restricting access to data and protecting it from unauthorized modification. This improves data integrity and reduces the likelihood of bugs and errors.
Polymorphism: OOP supports polymorphism, which allows objects of different classes to be treated uniformly if they exhibit similar behavior. This enables code to be more flexible and adaptable to different types of objects.
Scalability: OOP facilitates code scalability by providing a structured and organized approach to software development. Classes and objects can be easily extended and modified to accommodate changing requirements and evolving software projects.
31. What is a
class in Python? How do you define and instantiate a class?
A class in
Python is a blueprint for creating objects. It defines the attributes (data)
and methods (behavior) that objects of the class will have. Classes are defined
using the class keyword, and objects are instantiated using the class
name followed by parentheses.
Example:
class Car: #Defining the Class
def __init__(self, make, model):
self.make = make
self.model = model
my_car =
Car(“Toyota”, “Camry”)
32. What are
attributes and methods in a class? How do you access them?
Attributes and Methods in a Class:
In a Python class, attributes are variables that store data associated with objects of the class, while methods are functions that define behavior or operations that can be performed by the objects. They collectively represent the state and behavior of objects within the class.
Attributes: Attributes are variables bound to instances of a class. They represent the state of an object and hold data associated with that object. Attributes are defined within the class and accessed using dot notation (object.attribute).
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
my_car = Car(“Toyota”, “Camry”)
print(my_car.make) # Accessing the ‘make’ attribute
print(my_car.model)
# Accessing the ‘model’ attribute
In the above example, make and model are attributes of the Car class. They are initialized using the __init__() method and accessed using dot notation.
Methods: Methods are functions defined within a class that define the behavior of the class and its objects. They operate on the data stored in attributes and can perform various actions or computations. Methods are defined within the class using the def keyword.
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def start(self):
print(f”The {self.make}
{self.model} is starting.”)
my_car = Car(“Toyota”, “Camry”)
my_car.start() # Calling the ‘start’ method
In the above example, start() is a method of the Car class. It prints a message indicating that the car is starting. Methods are accessed using dot notation (object.method()).
Accessing Attributes and Methods: Attributes and methods of a class are accessed using dot notation, where the object name is followed by a dot . and the attribute or method name.
print(my_car.make) # Accessing the ‘make’ attribute
print(my_car.model) # Accessing the ‘model’ attribute
my_car.start() # Calling the ‘start’ method
33. What is a constructor in Python? How do you define and use it?
Answer: A constructor is a special method (__init__()) used for initializing new objects of a class. It is automatically called when a new object is instantiated. Constructors are used to initialize instance variables and perform any necessary setup for the object.
Example:
class
Person:
def __init__(self, name, age): #This is
called constructor
self.name = name
self.age = age
person1 = Person(“Alice”, 30)
The
__init__ method is the constructor. It takes three parameters: self, name, and
age. The name and age parameters are used to initialize the newly created
object.
self.name and self.age are instance attributes that are set to the values passed to the constructor when a new Person object is created.
34. What is inheritance in Python? How do you create a subclass?
Inheritance in Python: Inheritance is a fundamental concept in object-oriented programming (OOP) that allows a new class (subclass) to inherit attributes and methods from an existing class (superclass).
It enables code reuse and the creation of hierarchical relationships between classes. Inheritance allows subclasses to extend or modify the behavior of the superclass, promoting modularity and extensibility in code.
Creating
a Subclass:
To create a subclass in Python, you define a new class that inherits from an existing class. The subclass inherits all attributes and methods of the superclass, allowing it to reuse the functionality provided by the superclass. Subclasses can also define their own attributes and methods, extending or modifying the behavior inherited from the superclass.
Here’s the syntax for creating a subclass in Python:
class
SubclassName(SuperclassName):
# Subclass attributes and methods go here
Pass
In this syntax:
SubclassName is the name of the subclass being defined.
SuperclassName is the name of the superclass from which the subclass inherits
35. What is method overriding in Python? How do you override methods in a subclass?
Method
Overriding in Python:
Method overriding is a feature of object-oriented programming that allows a subclass to provide a specific implementation of a method that is already defined in its superclass. When a method is overridden in a subclass, the subclass provides a new implementation of the method that replaces the implementation inherited from the superclass. This allows subclasses to customize or extend the behavior of methods inherited from the superclass.
How to Override Methods in a Subclass:
To override a method in a subclass in Python, you define a method with the same name as the method in the superclass. The method signature (name and parameters) must match that of the superclass method to ensure proper overriding. When an instance of the subclass calls the overridden method, the new implementation provided by the subclass is executed instead of the inherited implementation from the superclass.
Here’s the general process for method overriding in Python:
- Define a subclass that inherits from a superclass.
- Define a method with the same name as a method in the superclass.
- Provide a new implementation of the method in the subclass.
class
Animal:
def make_sound(self):
print(“Generic animal sound”)
class
Dog(Animal):
def make_sound(self): # method overriding
print(“Woof woof”)
class
Cat(Animal):
def make_sound(self): # method overriding
print(“Meow”)
# Creating
instances of Dog and Cat
dog = Dog()
cat = Cat()
# Calling
the make_sound method for each instance
dog.make_sound() # Output: Woof woof
cat.make_sound() # Output: Meow
36. What is encapsulation in Python?
Encapsulation is the concept of bundling data (attributes) and methods (behavior) that operate on the data into a single unit (class). It restricts access to the data to prevent accidental modification and ensures data integrity by controlling access through methods.
Private members of a class are denoted by prefixing the name with two underscores __, and they cannot be accessed directly from outside the class. However, it’s worth noting that Python does not enforce strict encapsulation as seen in some other languages; it’s based more on a convention (a guideline), and it can be bypassed if necessary.
37. Give an example
of Encapsulation in Python?
class
BankAccount:
def __init__(self, account_number,
balance=0):
self.__account_number =
account_number # Private attribute
self.__balance = balance # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
self.__show_balance()
else:
print(“Deposit amount must be positive”)
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
self.__show_balance()
else:
print(“Invalid withdrawal amount”)
def __show_balance(self): # Private method
print(f”Balance: {self.__balance}”)
#
Creating an instance of BankAccount
account = BankAccount(“123456789”, 1000)
#
Accessing the public methods
account.deposit(500)
account.withdraw(200)
#
The following attempts to access private attributes will result in an
AttributeError
try:
print(account.__balance)
except
AttributeError as e:
print(“This attribute is
private”)
# The correct way to interact with the
balance would be through the deposit and withdraw methods
In this example:
The BankAccount class has two private
attributes: __account_number and __balance. These attributes are meant to be
accessed and modified only through the class’s methods, like deposit, withdraw,
and the private method __show_balance.
The methods deposit
and withdraw allow controlled access to the __balance attribute, ensuring that
only valid operations can be performed (e.g., no direct modification to the
balance, no withdrawals that exceed the available balance).
The __show_balance
method is private and is used internally by the class to display the
balance. It cannot be called from outside the class directly.
38. What is polymorphism in Python? How does it work?
Polymorphism
in Python:
Polymorphism
is a fundamental concept in object-oriented programming (OOP) that allows
objects of different classes to be treated as objects of a common superclass.
It enables a single interface to represent multiple types of objects and allows
methods to behave differently based on the object’s type or class. Polymorphism
promotes flexibility, extensibility, and code reusability by enabling code to
work with objects of different types without needing to know their specific
class.
How Polymorphism Works in Python:
Polymorphism
in Python is achieved through method overriding and method overloading.
class
Animal:
def make_sound(self):
print(“Generic animal sound”)
class Dog(Animal):
def make_sound(self):
print(“Woof woof”)
class Cat(Animal):
def make_sound(self):
print(“Meow”)
# Polymorphism in action
def
animal_sound(animal):
animal.make_sound()
dog = Dog()
cat = Cat()
animal_sound(dog) # Output: Woof woof
animal_sound(cat) # Output: Meow
39. How do you access class variables and instance variables in Python?
Class variables are shared among all instances of a class and are accessed using the class name or instance name. Instance variables are unique to each instance and are accessed using the instance name (self).
Example:
class MyClass:
class_var = 10 # Class variable
def __init__(self, instance_var):
self.instance_var = instance_var # Instance variable
print(MyClass.class_var) # Accessing class variable
obj = MyClass(20)
print(obj.instance_var) # Accessing instance variable
40. What is NumPy in Python? How is it used?
Answer: NumPy is a library in Python used for numerical computing. It provides support for multidimensional arrays and matrices, along with mathematical functions to operate on these arrays efficiently.
Example:
import numpy as np
# Create a NumPy array
arr = np.array([1, 2, 3, 4, 5])
print(arr) # Output: [1 2 3 4 5]
41. What are Pandas in Python? How are they used?
Pandas is a library in Python used for data manipulation and analysis. It provides data structures like DataFrame and Series, along with functions to manipulate and analyze data efficiently.
42. What is a DataFrame in Pandas? Provide an example.
A DataFrame is a 2-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or SQL table.
import pandas as pd
# Create a DataFrame
data = {‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’], ‘Age’: [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
43. What is Matplotlib in Python?
Matplotlib is a popular plotting library in Python used for creating static, animated, and interactive visualizations. It provides a wide range of plotting functionalities, allowing users to create various types of plots such as line plots, scatter plots, bar plots, histograms, pie charts, and more. Matplotlib is highly customizable, providing users with control over the appearance and styling of their plots.
44. What are the main differences between NumPy arrays and Python lists?
The main differences between NumPy arrays and Python lists are as follows:
Homogeneity:
· NumPy arrays are homogeneous, meaning all elements in the array must have the same data type (e.g., integers, floats, strings).
· Python lists can contain elements of different data types.
Memory Efficiency:
· NumPy arrays are more memory-efficient compared to Python lists. They store data in contiguous memory blocks, allowing for faster access and manipulation of elements.
· Python lists store references to objects, which can lead to higher memory overhead and slower performance, especially for large datasets.
Performance:
· NumPy arrays provide faster numerical computations and operations compared to Python lists, especially for large datasets. This is because NumPy uses optimized, compiled C code under the hood.
· Python lists are slower for numerical computations and operations, as they are implemented in Python and do not have built-in support for vectorized operations.
Functionality:
· NumPy arrays offer a wide range of mathematical functions and operations for numerical computing, such as element-wise arithmetic operations, matrix multiplication, statistical functions, and linear algebra operations.
· Python lists provide basic functionality for storing and manipulating data but lack built-in support for numerical computations and operations.
Syntax and Usage:
· NumPy arrays are created using the np.array() function and support indexing, slicing, and element-wise operations.
· Python lists are created using square brackets [ ] and support indexing, slicing, appending, and extending.
Size and Shape:
· NumPy arrays have a fixed size and shape, which cannot be changed once created. However, elements can be added, removed, or modified within the array.
· Python lists have dynamic size and shape, allowing for the addition, removal, and modification of elements without restrictions.
45. What is a
generator in Python? And how do you create a generator function in Python?
In
Python, a generator is a special type of iterator, a function that allows you
to declare a function that behaves like an iterator, i.e., it can be used in a
for loop. Generators are written as regular functions but use the yield
statement whenever they want to return data.
Each time yield is called, the function generates a new value, which can be iterated over. This means that instead of returning all values at once (like a list) and holding them in memory, a generator produces one value at a time, which is much more memory-efficient for large datasets.
def
my_generator():
yield 1
yield 2
yield 3
gen = my_generator()
46. What is the difference between a generator function and a normal function?
The primary difference between a generator function and a normal function in Python lies in how they return data and manage their execution state. Here’s a breakdown of the key differences:
Normal Function:
Return Values: A normal function uses return statements to send back values. Once a return statement is executed, the function terminates and exits, returning control back to the caller. If the function needs to return multiple values, it usually does so all at once, often in a list, tuple, or another container.
State: Normal functions do not maintain state between calls. Each time you call a function, it starts with a fresh state, performs its operations, and exits. If you call the function again, it does not remember any values from the previous calls unless those values are stored outside the function (e.g., in global variables or closures).
Use Case: Ideal for calculations and tasks that produce a single result or a collection of results at once.
Generator Function:
Yield Statements: A generator function uses yield statements to return data. Unlike return, yield provides a value to the code that’s calling the generator function but then pauses the function’s execution, saving its state for later. The next time the generator is called, it resumes where it left off, immediately after the last yield.
State: Generator functions maintain state between yields. They remember where they were in the execution process, including the values of variables, and resume from there the next time the __next__() method of the generator object is called.
Lazy Evaluation: Generators produce values one at a time and only on demand, which is known as lazy evaluation. This can lead to significant memory savings when working with large datasets or infinite sequences, as values are generated and consumed sequentially rather than being stored in memory.
Use Case: Ideal for iterating over sequences of data that are too large to fit in memory all at once, or when the complete result set is not needed immediately.
47. What is the advantage of using generators over lists?
The advantage of using generators over lists in Python lies primarily in memory efficiency and performance optimization:
Memory Efficiency:
·
Generators
produce values on-the-fly as they are needed, rather than storing all values in
memory at once like lists. This is particularly beneficial when dealing with
large datasets or infinite sequences, as generators conserve memory by
generating values dynamically.
·
Lists
store all elements in memory, which can lead to high memory consumption,
especially for large datasets. In contrast, generators produce values one at a
time, allowing for efficient memory usage.
Lazy
Evaluation:
·
Generators
support lazy evaluation, meaning they generate values only when they are
requested. This enables efficient processing of data without the need to load
the entire dataset into memory.
·
Lists,
on the other hand, eagerly evaluate all elements when created, consuming memory
even if not all elements are immediately needed.
Performance
Optimization:
·
Generators
can lead to improved performance in scenarios where only a subset of values is
required or when processing large datasets incrementally.
·
Iterating
over a generator typically requires fewer computational resources compared to
iterating over a list, especially for operations that involve complex
calculations or transformations.
Suitability
for Infinite Sequences:
·
Generators
are well-suited for representing and processing infinite sequences or streams
of data, such as sensor readings, event streams, or numerical sequences.
· List cannot represent infinte sequence due to their finite size and memory limitation.
48. Explain the concept of decorators in Python. And what are some common use cases for decorators in Python?
Decorators in Python are a powerful and expressive tool for modifying or enhancing the behavior of functions or methods without permanently modifying their actual code. They are defined with functions, which wrap another function or method to add functionality before and/or after the target function runs, without changing the target function’s behavior directly.
A decorator in Python is a callable that takes another function or method as an argument and returns a function or method. The basic syntax for a decorator involves the @ symbol followed by the decorator function name, placed above the definition of the function to be decorated.
# Define the decorator
def simple_decorator(func):
def wrapper():
print(“Something is happening before the function is called.”)
func()
print(“Something is happening after the function is called.”)
return wrapper
# Use the decorator
@simple_decorator
def say_hello():
print(“Hello!”)
# Call the decorated function
say_hello()
When you run say_hello(), the output will be:
Output:
Something is happening before the function is called.
Hello!
Something is happening after the function is called
49. Explain the concept of class-based decorators in Python.
Class-based decorators in Python are implemented using classes instead of functions. They typically define __init__() and __call__() methods to initialize the decorator with arguments and execute the decoration process.
50. Explain the concept of method decorators in Python.
Method decorators in Python are used to modify or enhance the behavior of class methods. They are similar to function decorators but are applied to methods within a class.
51. What are lambda functions in Python?
Lambda functions, also known as anonymous functions, are small, inline functions defined using the lambda keyword. They can have any number of arguments but can only contain a single expression. Lambda functions are typically used for short, simple operations where defining a named function is unnecessary.
Here’s an example of a lambda function that calculates the square of a number:
square =
lambda x: x ** 2
print(square(5))
# Output: 25
52. Explain the difference between lambda functions and regular functions in Python.
The difference between lambda functions and regular functions in Python lies primarily in their syntax, purpose, and capabilities:
Syntax:
Lambda functions are defined using the lambda keyword, followed by a list of parameters, a colon (:), and a single expression. They can be written in a single line and do not require a return statement.
Regular functions are defined using the def keyword, followed by the function name, a list of parameters enclosed in parentheses, a colon (:), and one or more statements inside the function body. They can span multiple lines and include a return statement to return a value.
Purpose:
Lambda functions are typically used for short, simple operations where defining a named function is unnecessary or inconvenient. They are often used as anonymous functions passed as arguments to higher-order functions or used inline in expressions.
Regular functions are used for more complex or reusable code that requires multiple statements or computations. They are named, making them easier to identify and debug, and can be called from anywhere within the scope where they are defined.
Readability and Maintainability:
Lambda functions are concise and can make code more compact, especially when used with higher-order functions like map(), filter(), and sorted(). However, they can sometimes sacrifice readability and may be harder to understand for those unfamiliar with lambda syntax.
Regular functions provide better readability and maintainability, as they can have descriptive names and meaningful docstrings. They are easier to debug and refactor, making them preferable for larger or more complex codebases.
Scope and Name Binding:
Lambda functions are anonymous and are not bound to a specific name or scope. They are often used as throwaway functions within a limited context.
Regular functions are named entities that can be assigned to variables, passed as arguments, or returned from other functions. They have their own scope and namespace, allowing for better organizarion and reuse of code.
53. How do you use lambda functions with higher-order functions like map(), filter(), and reduce()?
Lambda functions can be used with higher-order functions to apply transformations, filter elements, or perform reductions on iterables.
For example:
# Using
map() with lambda function
nums = [1,
2, 3, 4, 5]
squared_nums = map(lambda x: x ** 2, nums)
# Using
filter() with lambda function
even_nums = filter(lambda x: x % 2 == 0, nums)
# Using
reduce() with lambda function (in Python 3, it’s in functools)
from
functools import reduce
product = reduce(lambda
x, y: x * y, nums)
54. Provide an example of using lambda functions to sort a list of tuples based on a specific element.
Here’s an example of sorting a list of tuples based on the second element using a lambda function:
tuples =
[(1, 2), (3, 1), (5, 3)]
sorted_tuples
= sorted(tuples, key=lambda x: x[1])
print(sorted_tuples)
# Output: [(3, 1), (1, 2), (5, 3)]
55. Explain the limitations of lambda functions in Python.
Lambda functions in Python are limited to a single expression and cannot contain statements or multiple lines of code. They are primarily used for simple, inline operations and are not suitable for complex logic or computations.
56. What are comprehensions in Python? Explain their purpose and benefits.
Comprehensions are concise and expressive constructs in Python for creating collections (lists, dictionaries, sets) by applying transformations or filters to existing iterables. They provide a more readable and efficient way to generate sequences compared to traditional loops.
Here’s an example of a list comprehension that squares each element of a list:
nums = [1,
2, 3, 4, 5]
squared_nums
= [x ** 2 for x in nums]
print(squared_nums) # Output: [1, 4, 9, 16, 25]
57. Explain the concept of conditional expressions in list comprehensions.
Conditional expressions in list comprehensions allow you to filter elements based on a condition. Elements satisfying the condition are included in the resulting list comprehension.
For example:
nums = [1,
2, 3, 4, 5]
even_nums =
[x for x in nums if x % 2 == 0]
print(even_nums) # Output: [2, 4]
58. How can you use nested list comprehensions in Python?
Nested list comprehensions allow you to create lists of lists or perform multiple transformations in a single expression.
For example:
matrix =
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened_matrix
= [num for row in matrix for num in row]
print(flattened_matrix) # Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
59. What are dictionary comprehensions in Python? Provide an example.
Dictionary comprehensions are similar to list comprehensions but create dictionaries instead of lists. They use key-value pairs and the {key: value} syntax.
For example:
nums = [1, 2, 3, 4, 5]
squared_dict = {x: x ** 2 for x in nums}
print(squared_dict) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
60. Explain the concept of set comprehensions in Python.
Set comprehensions create sets by applying transformations or filters to existing iterables. They use curly braces {} and produce unique elements.
For example:
nums = [1, 2, 3, 4, 5]
squared_set = {x ** 2 for x in nums}
print(squared_set) # Output: {1, 4, 9, 16, 25}
61. What are map, filter, and reduce functions in Python?
map(): The map() function applies a given function to each item of an iterable (such as a list) and returns an iterator of the results.
filter(): The filter() function applies a given function to each item of an iterable and returns an iterator containing only the items for which the function returns true.
reduce(): The reduce() function (in Python 2, it was a built-in function but moved to functools module in Python 3) applies a given function to the first two items of an iterable, then applies the same function to the result and the next item, and so on, until all items are processed and a single value is returned.
62. Provide an example of using map() to double each element of a list.
nums = [1,
2, 3, 4, 5]
doubled_nums
= list(map(lambda x: x * 2, nums))
print(doubled_nums) # Output: [2, 4, 6, 8, 10]
63. Provide an example of using filter() to filter out even numbers from a list.
nums = [1,
2, 3, 4, 5]
odd_nums =
list(filter(lambda x: x % 2 != 0, nums))
print(odd_nums) # Output: [1, 3, 5]
64. How can you use map() and filter() functions together in Python?
You can chain map() and filter() functions together using generator expressions or list comprehensions to perform multiple transformations and filtering in a single expression.
An example of chaining map() and filter() functions together.
nums = [1,
2, 3, 4, 5]
result =
list(map(lambda x: x * 2, filter(lambda x: x % 2 != 0, nums)))
print(result) # Output: [2, 6, 10]
65. What is the purpose of the os module in Python? Provide examples of its usage.
The os module provides functions for interacting with the operating system, such as manipulating files and directories, working with paths, and accessing environment variables.
Example:
import os
# Get the
current working directory
cwd =
os.getcwd()
print(“Current working directory:”, cwd)
# List files
and directories in a directory
files =
os.listdir(cwd)
print(“Files and
directories:”, files)
66. Explain the difference between os.path.join() and os.path.abspath() functions.
os.path.join() is used to concatenate one or more path components intelligently, creating a valid path string.
os.path.abspath() returns the absolute path of a given path string.
67. What is the purpose of the sys module in Python? Provide examples of its usage.
The sys module provides access to some variables used or maintained by the Python interpreter and to functions that interact strongly with the interpreter.
Example:
import sys
# Get the
Python version
print(“Python version:”, sys.version)
# Get the
command line arguments
print(“Command line arguments:”, sys.argv)
68. What is the purpose of the datetime module in Python? Provide examples of its usage.
The datetime module provides classes for manipulating dates and times in both simple and complex ways.
Example:
import datetime
# Get the
current date and time
now =
datetime.datetime.now()
print(“Current date and time:”, now)
# Format a
date as a string
formatted_date
= now.strftime(“%Y-%m-%d %H:%M:%S”)
print(“Formatted date:”, formatted_date)
69. Explain the purpose of the random module in Python. Provide examples of its usage.
The random module provides functions for generating random numbers, selecting random elements, and shuffling sequences.
Example:
import random
# Generate a
random integer between 1 and 10
random_num =
random.randint(1, 10)
print(“Random number:”, random_num)
# Shuffle a
list
my_list =
[1, 2, 3, 4, 5]
random.shuffle(my_list)
print(“Shuffled list:”, my_list)
70. What are Boolean values used for in Python?
Boolean values in Python are used to represent truth values, typically denoting whether a condition is true or false. They are fundamental in controlling the flow of program execution through conditional statements, such as `if`, `elif`, and `else`.
Boolean values are also used in boolean operations such as `and`, `or`, and `not`, allowing for logical comparisons and combinations of conditions. These operations are commonly used for decision-making and controlling program behavior based on certain conditions.
Moreover, boolean values are returned by comparison operators like `==` (equal), `!=` (not equal), `>`, `<`, `>=`, `<=`, etc., when comparing two values.
In summary,
boolean values serve as the backbone of conditional logic and decision-making
in Python programs, enabling developers to create dynamic and responsive
applications by evaluating and reacting to different conditions.
71. Explain the difference between a “for” loop and a “while” loop?
For Loop: A “for” loop is used when the number of iterations is known before the loop starts. Its syntax is designed to include the initialization of the loop variable, the condition for the loop to continue, and the increment or decrement operation—all in one line.
This makes “for” loops ideal for iterating over sequences (like lists, arrays, or strings) or executing a loop a specific number of times. The general syntax in many programming languages looks something like this:
While Loop: A “while” loop, on the other hand, is used when the number of iterations is not known before the loop starts. The loop continues as long as a specified condition is true and stops once the condition becomes false. The “while” loop starts with the condition, and the loop’s control variables (if any) need to be initialized before the loop starts and modified during the loop’s execution.
72. Can you convert a “for” loop into an equivalent “while” loop? Provide an example.
For Loop Example
for i in range(1, 6): # Note: range(1, 6) generates numbers from 1 to 5
print(i)
This “for” loop starts at 1 and goes up to (but does not include) 6, effectively counting from 1 to 5.
Output:
1
2
3
4
5
Equivalent While Loop
To convert this “for” loop into a “while” loop, we need to manually handle the initialization of the loop variable (i), the condition to continue the loop, and the increment of the loop variable.
i = 1 # Initialization outside the loop
while i < 6: # Condition to continue
print(i)
i += 1 # Increment inside the loop
Output:
1
2
3
4
5
In this “while” loop:
· The loop variable i is initialized to 1 before the loop starts.
· The loop continues as long as i is less than 6, mirroring the range(1, 6) condition in the “for” loop.
· Within the loop body, i is incremented by 1 after each iteration, ensuring the loop will eventually terminate when i reaches 6.
This “while” loop behaves identically to the original “for” loop, printing numbers from 1 to 5. This conversion process demonstrates the manual handling of loop control variables in “while” loops that is implicitly managed in “for” loops.
73. What are *args and *kwargs?
To pass a variable number of arguments to a function in Python, use the special syntax *args and **kwargs in the function specification.
· *args (Non-Keyword Arguments): *args allows a function to accept any number of positional arguments beyond those explicitly defined. The arguments are accessed as a tuple within the function. It’s used when you’re not sure how many arguments might be passed to your function, allowing for flexibility.
Example of *args:
def add_numbers(*args):
return sum(args)
#Calling the function
print(add_numbers(1, 2, 3)) # Outputs: 6
print(add_numbers(1, 2, 3, 4, 5)) # Outputs: 15
· **kwargs (Keyword Arguments): **kwargs allows a function to accept any number of keyword arguments (arguments provided in the form of key-value pairs). The keyword arguments are accessed as a dictionary within the function. It’s used when you want to handle named arguments in your function dynamically.
Example of *kwargs:
def introduce_yourself(**kwargs):
for key, value in kwargs.items():
print(f”{key}: {value}”)
#Calling the function
introduce_yourself(Name=”John”, Age=25, Occupation=”Engineer”)
#Output
Name: John
Age: 25
Occupation: Engineer
74. How do you
create a NumPy array, and what are the different ways to do so?
Here are several common ways to
create NumPy arrays:
1. From a Python List or Tuple
You can create a NumPy array from a
Python list or tuple by using the np.array() function.
import numpy as np
# From a list
my_array = np.array([1, 2, 3, 4,
5])
print(my_array)
# From a tuple
my_tuple_array = np.array((6, 7, 8,
9, 10))
print(my_tuple_array)
2.
Using Built-in NumPy Functions
NumPy
offers a variety of functions to create arrays for specific needs:
Zeros:
Creates an array filled with zeros.
zeros_array
= np.zeros((2, 3)) # Create an array of
zeros with shape (2, 3)
Ones:
Creates an array filled with ones.
ones_array
= np.ones((3, 2)) # Create an array of
ones with shape (3, 2)
Arange:
Creates arrays with regularly incrementing values.
range_array
= np.arange(10) # Similar to Python’s
range but returns a NumPy array
Linspace:
Creates arrays with a specified number of elements, and spaced equally between
the specified beginning and end values.
linspace_array
= np.linspace(0, 1, 5) # Create an array
of five values evenly spaced between 0 and 1
Random:
NumPy’s random module can generate arrays of random numbers.
random_array
= np.random.rand(2, 3) # Create an array
of the given shape and populate it with random samples from a uniform
distribution over [0, 1).
3.
With a Specified Data Type
You
can specify the desired data type at creation using the dtype keyword.
int_array
= np.array([1, 2, 3, 4], dtype=’int32′)
4.
Creating Multidimensional Arrays
Passing
lists of lists (or tuples) to np.array() creates multidimensional arrays.
multi_array
= np.array([[1, 2, 3], [4, 5, 6]])
5.
Using np.empty
Creates
an array without initializing its values to any particular value. It’s faster
than np.zeros or np.ones for large arrays if you plan to fill the entire array
later.
empty_array
= np.empty((3, 3)) # Note: The values
will be whatever happens to already be at that memory location
Each
of these methods serves different purposes and can be used based on the
specific needs of your program or analysis.
75. Explain
the difference between a NumPy array and a Python list.
NumPy arrays and Python lists are
both used for storing collections of data in Python, but they serve different
purposes and have distinct differences in terms of functionality, performance,
and use cases. Here are the key differences between NumPy arrays and Python
lists:
1. Data Homogeneity
NumPy Arrays: Designed to store
elements of a single data type. This homogeneity allows for more efficient
storage and operations, as NumPy can optimize memory usage and processing for
the specific type of data stored.
Python Lists: Can store elements of
different data types within the same list. Lists are more flexible in terms of
the types of data they can contain, but this flexibility comes at the cost of
performance and memory efficiency.
2. Performance
NumPy Arrays: Generally offer
better performance for numerical operations because they are implemented in C
and use fixed types. They are specifically optimized for numerical and
array-oriented computing, which makes operations on NumPy arrays much faster
for large datasets.
Python Lists: Less efficient for
numerical operations due to their dynamic nature and the overhead of handling
different data types. Operations on lists, especially large ones, can be
significantly slower compared to equivalent operations on NumPy arrays.
3. Functionality
NumPy Arrays: Come with a wide
range of mathematical and array operations built-in, such as linear algebra
operations, statistical operations, and more. They support vectorization, which
allows for operations to be applied directly to the arrays without the need for
explicit loops.
Python Lists: Lack the extensive
set of operations for mathematical processing. Operations typically require
explicit loops or the use of additional libraries like math or comprehensions.
4. Memory Usage
NumPy Arrays: More memory efficient
for storing large data sets of homogeneous data. The homogeneity of data and
fixed types allow NumPy to use less memory and perform optimizations not
possible with heterogeneous data.
Python Lists: Consume more memory
for large datasets, especially when storing mixed data types, due to the
overhead associated with the flexibility of lists.
76. How can
you handle missing data in a NumPy array?
Using numpy.nan Functions
NumPy provides several functions
that are nan-safe, meaning they ignore nan values in their computations. Some
of these functions include np.nansum(), np.nanmean(), np.nanmedian(),
np.nanvar(), etc., which can calculate the sum, mean, median, and variance,
respectively, while ignoring nan values.
Example:
# Replace missing values with the
mean of the remaining values
mean_val = np.nanmean(data)
data[np.isnan(data)] = mean_val
77. What is
the purpose of the reshape method in NumPy, and how do you use it?
The
reshape method in NumPy is used to change the shape of an array without
changing its data. Essentially, it allows you to reorganize the array into a
new shape, making it a powerful tool for data manipulation and preparation. The
new shape must be compatible with the original size of the array, meaning the
total number of elements must remain the same.
Example
1: Reshaping a 1D array to a 2D array
import
numpy as np
#
Creating a 1D array of 12 elements
arr
= np.arange(12)
print(“Original
array:\n”, arr)
#
Reshaping it to a 2D array of 3 rows and 4 columns
reshaped_arr
= arr.reshape((3, 4))
print(“Reshaped array:\n”, reshaped_arr)
78. How do you
slice and index NumPy arrays, and how does it differ from Python lists?
Slicing and indexing in NumPy
arrays offer more powerful and flexible ways to access and manipulate array
data compared to Python lists. While Python lists support basic slicing and
indexing, NumPy extends these capabilities, especially for multidimensional
arrays.
Indexing in Python Lists:
Single Element: Access a single element
by its position.
Slices: Access a subset of the list using
the slice notation [start:stop:step].
my_list = [1, 2, 3, 4, 5]
print(my_list[2]) # Output: 3
print(my_list[1:4]) # Output: [2, 3, 4]
Indexing and Slicing in NumPy
Arrays:
NumPy arrays extend the concept of
slicing and indexing by allowing multidimensional array slicing and providing
more options for indexing.
Single Element: Similar to lists, but
for multidimensional arrays, you can use a tuple of indices.
Slices: Works like list slicing but can be
applied to multiple dimensions [start:stop:step, start:stop:step].
Example: Slicing and Indexing NumPy
Arrays
import numpy as np
# Creating a 2D NumPy array
arr = np.array([[1, 2, 3], [4, 5,
6], [7, 8, 9]])
# Single element access
print(arr[0, 1]) # Output: 2
79. Explain
the use of the axis parameter in NumPy’s aggregation functions?
When you perform an aggregation
function on a NumPy array and specify an axis, the function is applied along
that axis, collapsing the array along that axis and reducing its
dimensionality.
·
axis=None
(default): The aggregation function will be applied to the entire array,
treating it as a flat array. No axis is collapsed, and a single scalar value is
returned.
·
axis=0:
The function is applied vertically down the array’s rows. For a 2D array, this
means each column’s values will be aggregated.
·
axis=1:
The function is applied horizontally across the array’s columns. For a 2D
array, this means each row’s values will be aggregated.
Examples: Consider a 2D array:
import numpy as np
arr = np.array([[1, 2, 3], [4, 5,
6], [7, 8, 9]])
# Sum of the entire array
print(np.sum(arr, axis=None)) # Output: 45
# Sum down the rows (collapsing
rows)
print(np.sum(arr, axis=0)) # Output: [12 15 18]
# Sum across the columns
(collapsing columns)
print(np.sum(arr, axis=1)) # Output: [ 6 15 24]
80. How can
you perform linear algebra operations using NumPy?
NumPy
provides a comprehensive set of functions for linear algebra operations through
its numpy.linalg module. These functions allow you to perform various linear
algebra tasks, such as vector and matrix multiplication, finding determinants,
solving linear systems, eigenvalues, and eigenvectors, among others. Here’s how
you can perform some common linear algebra operations using NumPy:
1.
Vector
and Matrix Multiplication: For
dot products, matrix multiplication, and other related operations, you can use np.dot()
2.
Determinant
of a Matrix:
You can use np.linalg.det() to calculate the determinant of a matrix.
3.
Inverse
of a Matrix: To
find the inverse of a square matrix, use np.linalg.inv()
4.
Solve
Linear Systems: To
solve a linear system of equations Ax=b, use np.linalg.solve().
5.
Eigenvalues
and Eigenvectors: To
find the eigenvalues and eigenvectors of a matrix, use np.linalg.eig().
81. Describe
how vectorization works in NumPy and its benefits over traditional looping
methods.
Benefits of Vectorization Over
Traditional Looping
Performance: Vectorized operations
are significantly faster than their equivalent operations implemented using
Python loops. The performance gain comes from both the use of efficient
low-level code and the reduction of overhead associated with Python function
calls and loops.
Simplicity and Readability: Vectorized operations
on arrays often result in simpler and more concise code. Operations that might
require several lines of code with loops can typically be performed with a
single line of vectorized code, making the code easier to read and maintain.
Consistency and Scalability: Vectorized code behaves
consistently across different sizes of data sets, making it easier to write
scalable and maintainable code. As data sets grow in size, vectorized
operations ensure that code performance degrades gracefully and predictably.
Example of Vectorization vs.
Traditional Looping
Consider the task of adding two
arrays element-wise:
Traditional Looping
import numpy as np
a = np.array([1, 2, 3, 4])
b = np.array([5, 6, 7, 8])
result = np.empty_like(a)
for i in range(len(a)):
result[i] = a[i] + b[i]
print(result)
Vectorized Approach
result = a + b
print(result)
In the vectorized approach, the
addition operation is applied to all elements of the arrays simultaneously,
leading to cleaner, more readable code and better performance.
82. How do you
read an Excel, CSV file into a Pandas Data Frame?
Reading a CSV File: To read a CSV
(Comma-Separated Values) file, you use the pd.read_csv() function.
import pandas as pd
# Assuming ‘data.csv’ is in your
current directory
df_csv = pd.read_csv(‘data.csv’)
Reading an Excel File: To read an Excel file,
you use the pd.read_excel() function.
import pandas as pd
# Assuming ‘data.xlsx’ is in your
current directory and contains a sheet named ‘Sheet1’
df_excel =
pd.read_excel(‘data.xlsx’, sheet_name=’Sheet1′)
83. Explain
the difference between a Series and a DataFrame in Pandas.
Dimensionality: The most fundamental
difference is that a Series is one-dimensional, while a DataFrame is
two-dimensional.
Data Structure: A Series can be thought
of as a single column of data, with an index. A DataFrame, on the other hand,
represents a rectangular table of data and contains an ordered collection of
columns, each of which can be a different value type.
Usage: Series are suited to handling
individual data columns, while DataFrames are better for dealing with multiple
data columns organized in a tabular form.
In practice, DataFrames and Series
are often used together, as DataFrames consist of multiple Series objects that
you can manipulate independently or in conjunction.
85. How can
you handle missing data in a Pandas DataFrame?
Dropping Missing Data: The dropna() method
allows you to drop rows or columns containing missing data.
Drop Rows with Any Missing Values:
By default, dropna() drops rows.
Filling Missing Data: The fillna() method
allows you to replace missing values with a specified value or method.
· Fill with a Specific Value: df.fillna(0)
·
Forward
Fill:
Propagate non-null values forward.
df.fillna(method=’ffill’)
·
Backward
Fill:
Propagate non-null values backward
df.fillna(method=’bfill’)
· Fill with Column Mean: df.fillna(df.mean())
86. What are
Pandas groupby operations, and how would you use them?
When performing groupby operations
in Pandas, you can apply various aggregate functions to the grouped data. These
functions are used to compute summary statistics or aggregated values for each
group. Here are some of the commonly used aggregate functions:
1. mean(): Computes the mean of the
groups.
2. sum(): Calculates the sum of
group values.
3. size(): Returns the size of each
group (including NaN values).
4. count(): Counts non-NA/null
observations in the groups.
5. std() and var(): Compute the standard
deviation (std()) and variance (var()) for each group, respectively.
6. min() and max(): Find the minimum (min())
and maximum (max()) values within each group.
7. median(): Calculates the median of
each group.
87. How do you
pivot data in a DataFrame?
The pivot() function is used to
reshape the DataFrame based on column values. It takes three essential
arguments: index, columns, and values. The index and columns arguments
determine the new layout of the DataFrame, while the values argument specifies
which column to use to fill in the new DataFrame.
import pandas as pd
# Sample DataFrame
df = pd.DataFrame({
‘Date’: [‘2021-01-01’, ‘2021-01-02’, ‘2021-01-01’, ‘2021-01-02’],
‘City’: [‘New York’, ‘New York’, ‘Los Angeles’, ‘Los Angeles’],
‘Temperature’: [65, 66, 68, 70],
‘Humidity’: [56, 58, 60, 62]})
# Pivot the DataFrame
pivot_df = df.pivot(index=’Date’,
columns=’City’, values=’Temperature’)
print(pivot_df)
87. What
methods can you use to manipulate strings in a Pandas DataFrame?
Pandas
provides a comprehensive set of vectorized string functions, which are very
efficient for manipulating and processing data in Series and DataFrames. These
methods are accessed through the .str accessor, which you can use to apply
string functions on each element of a Series or DataFrame column. Here are some
commonly used string manipulation methods:
df[‘column’].str.lower() # Convert to lowercase
df[‘column’].str.upper() # Convert to uppercase
df[‘column’].str.title() # Convert to titlecase
df[‘column’].str.capitalize() # Capitalize first letter of the string
df[‘column’].str.split(‘ ‘) # Split strings around given
separator/delimiter
df[‘column’].str.strip() # Remove leading and trailing whitespaces
df[‘column’].str.lstrip() # Remove
leading whitespaces
df[‘column’].str.rstrip() # Remove
trailing whitespaces
df[‘column’].str.contains(‘sub’) # Returns a boolean indicating if the
substring is found
df[‘column’].str.startswith(‘prefix’) # Check if the string starts with a prefix
df[‘column’].str.endswith(‘suffix’) # Check if the string ends with a suffix
df[‘column’].str.replace(‘old’,
‘new’) # Replace occurrences of a
pattern/substring
df[‘column’].str.count(‘sub’) # Count occurrences of a substring
88. How do you
convert the index of a DataFrame into a column?
To
convert the index of a Pandas DataFrame into a column, you can use the
.reset_index() method. This method resets the index of the DataFrame to the
default integer index and optionally adds the old index as a new column.
#
Convert the index into a column
df_reset
= df.reset_index()
89. Explain
how to implement data filtration in Pandas.
Data
filtration in Pandas involves selecting rows from a DataFrame based on the
values of one or more columns. This is a common operation in data analysis and
can be performed in several ways, using boolean indexing, query methods, or
conditional selection. Here’s how to implement data filtration in Pandas:
#
Filter rows where age is greater than 30
filtered_df
= df[df[‘age’] > 30]
#
Select rows where ‘age’ > 30 and only the ‘name’ and ‘city’ columns
filtered_df
= df.loc[df[‘age’] > 30, [‘name’, ‘city’]]
#
Filter rows where ‘age’ > 30 and ‘city’ is ‘NY’
filtered_df
= df[(df[‘age’] > 30) & (df[‘city’] == ‘NY’)]
#
Filter rows where ‘name’ is either ‘Alice’ or ‘David’
filtered_df
= df[df[‘name’].isin([‘Alice’, ‘David’])]
90. What are the
different join in pandas?
1. Inner Join: An inner join combines
two DataFrames based on their common keys (the intersection of keys). Only the
rows that have matching values in both DataFrames are included in the result.
result = pd.merge(df1, df2,
on=’key’, how=’inner’)
2. Outer Join (Full Outer Join): An outer join returns
all rows from both DataFrames, matching up rows by keys where possible and
filling in missing values with NaNs. It essentially unionizes the keys.
result = pd.merge(df1, df2,
on=’key’, how=’outer’)
3. Left Join: A left join returns all
rows from the left DataFrame and the matched rows from the right DataFrame. The
result will have NaNs in places where the right DataFrame does not have
matching rows.
result = pd.merge(df1, df2,
on=’key’, how=’left’)
4. Right Join: A right join returns
all rows from the right DataFrame and the matched rows from the left DataFrame.
The result will have NaNs in places where the left DataFrame does not have
matching rows.
result = pd.merge(df1, df2,
on=’key’, how=’right’)
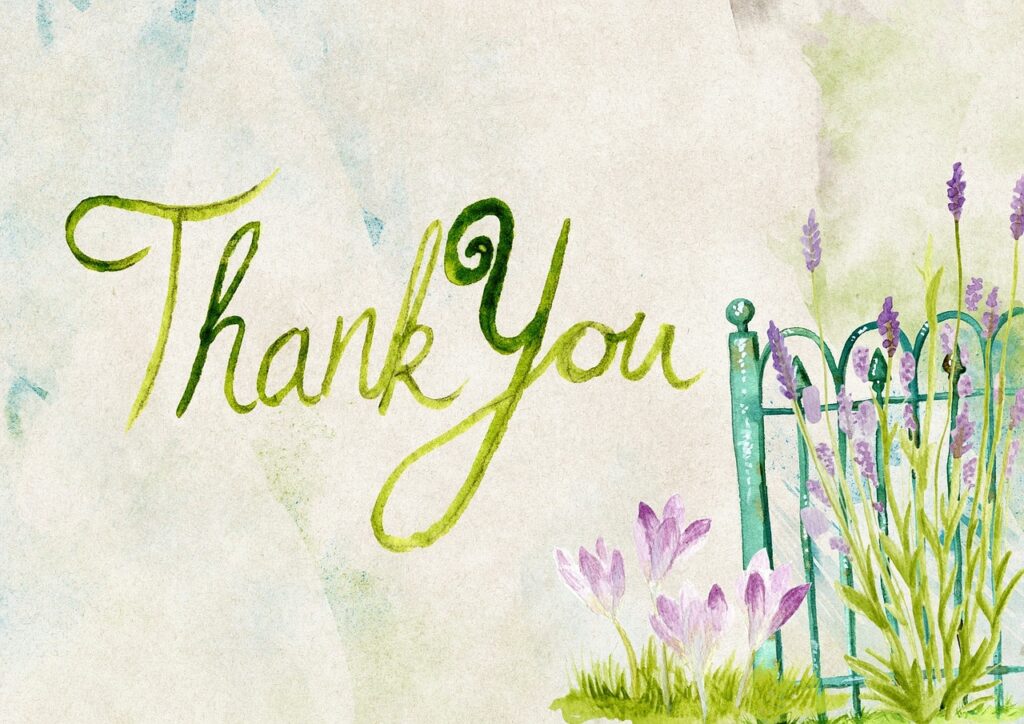